Building Like A Boss: SOLID Principles for Flawless Coding
In the ever-evolving world of software development, crafting clean, maintainable, and scalable code is paramount. While coding skills are essential, mastering certain design principles can elevate your coding game to a new level. Enter the SOLID principles- the five fundamental design principles that empower developers to write robust and maintainable code.
- Orna Ghosh
- 30 July, 2024
- 2 mins ago
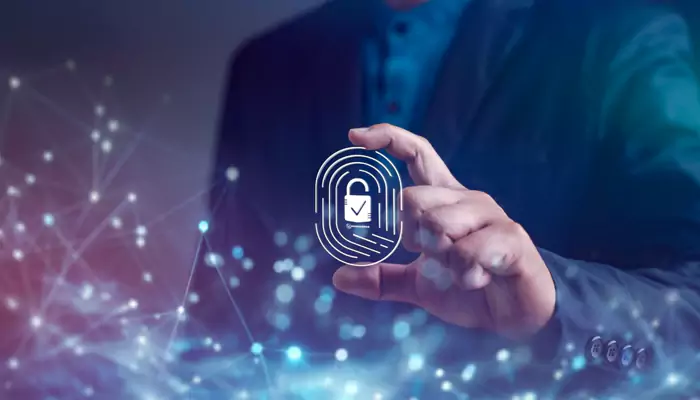
Building Like A Boss: SOLID Principles for Flawless Coding
In the ever-evolving world of software development, crafting clean, maintainable, and scalable code is paramount. While coding skills are essential, mastering certain design principles can elevate your coding game to a new level. Enter the SOLID principles- the five fundamental design principles that empower developers to write robust and maintainable code.
The SOLID acronym was coined by Robert C. Martin, a renowned software engineer and author. These principles aim to guide developers in writing clean, object-oriented code that promotes code reusability, reduces complexity, and minimizes the risk of future bugs.
Single Responsibility Principle (SRP)
Imagine a class that handles user authentication, data processing, and user interface logic. Sounds like a recipe for disaster, right? The Single Responsibility Principle advocates having a single, well-defined responsibility. It means a class should focus on one specific task or functionality. Benefits of adhering to SRP include:
When a class has a single responsibility, it's easier to understand, modify, and test. Imagine a single function in your class instead of wrestling with a complex code block handling multiple tasks.
Loosely coupled classes rely less on each other, making the codebase more modular and easier to work with.
Single-responsibility classes are more reusable in different contexts of your application.
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules) should be open for extension but closed for modification. It's like adding extensions to your house- the core structure remains intact, but you can easily add new features like a sunroom or a deck.
Your code is easily extensible to accommodate future requirements without breaking existing functionality.
Since you're not modifying existing code, the chance of introducing bugs gets minimized.
OCP encourages inheritance and interfaces to define behaviour and enable extension without modifying existing code.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle emphasizes that objects of a subclass should be replaceable with objects of its superclass (parent class) without altering the program's correctness. Imagine having a class for "Animals" with the method "make sound." A subclass for "Birds" should inherit this method and still produce a sound, albeit a chirp instead of a roar.
Subclasses can be used interchangeably with their parent class, promoting code reusability.
The code becomes more robust and predictable by ensuring subtypes behave as expected.
Adhering to LSP minimizes potential runtime errors due to unexpected behaviour of subclasses.
Interface Segregation Principle (ISP)
The Interface Segregation Principle suggests creating smaller, specialized interfaces instead of one large, general-purpose interface. Think of it like specialized tools for different tasks- you wouldn't use a hammer to drill a hole, would you?
Smaller interfaces offer developers more flexibility in choosing the functionalities they need for specific tasks.
Classes are coupled only to the functionality they utilize, minimizing unnecessary dependencies.
Smaller interfaces are easier to understand, document, and maintain.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle advocates depending on abstractions (interfaces) rather than concretions (specific implementations). Imagine you rely on a specific email-sending library. If the library changes its API, your code breaks. You can easily switch to a different library without affecting your code by relying on an interface for email-sending functionality.
Classes depend on abstractions, making them less dependent on specific implementations and fostering modularity.
Abstractions are easier to mock and test in isolation, leading to more robust and reliable code.
Changes to concrete implementations don't ripple through your codebase, making it more adaptable and easier to maintain.
By embracing the SOLID principles, you can elevate your coding skills to a new level.